[Swift] 스프트의 switch 의 fallthrough 및 where 구문 예제
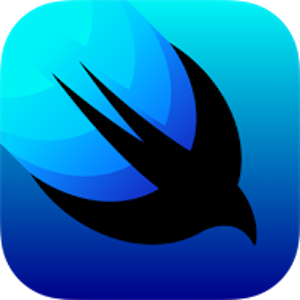
case문 구문결합하기
때로는 서로 다른 매칭(case)에 대해 동일한 코드가 실행되어야 하기도 한다. 이럴 때는 각각의 일치하는 경우들을 공통으로 실행될 구문과 묶을 수 있다. 예를 들어, 값이 0, 1, 또는 2일 경우에는 동일한 코드가 실행되도록 switch 구문을 수정할 수 있다.
let value = 1
switch (value) {
case 0, 1, 2:
print("zero, one or two")
case 3:
print("three")
case 4:
print("four")
case 5:
print("five")
default:
print("Integer out of range")
switch 구문에서 범위 매칭하기
switch 구문 안에 있는 case 구문에 범위 매칭을 구현할 수도 있다. 예를 들어, 다음의 switch 구문은 주어진 온도가 세 개의 범위 중에 매칭되는지를 검사한다.
let temperature = 83
switch (temperature) {
case 0...49:
print("Cold")
case 50...79:
print("Warm")
case 80...110:
print("Hot")
default:
print("Temperature out of range")
}
where 구문 사용하기
where 구문은 case 구문에 부가적인 조건을 추가하기 위해서 사용될 수 있다. 예를 들어, 다음의 코드는 값이 범위 조건에 일치하는지를 검사할 뿐만 아니라 그 숫자가 홀수인지 짝수인지도 검사한다.
let temperature = 54
switch (temperature) {
case 0...49 where temperature % 2 == 0:
print("Cold and even")
case 50...79 where temperature % 2 == 0:
print("Warm and even")
case 80...110 where temperature % 2 == 0:
print("Hot and even")
default:
print("Temperature out of range or odd")
}
fallthrough
스위프트에서는 case 구문 끝에 break를 쓸 필요가 없다. 다른 언어들과는 달리, 스위프트는 case 조건에 일치하면 자동으로 구문 밖으로 빠져나간다. fallthrough 구문을 사용하면 switch 구현부에 예외상황 효과를 주어, 실행 흐름이 그 다음의 case 구문으로 계속 진행하게 할 수 있다.
let temperature = 10
switch (temperature) {
case 0...49 where temperature % 2 == 0:
print("Cold and even")
fallthrough
case 50...79 where temperature % 2 == 0:
print("Warm and even")
fallthrough
case 80...110 where temperature % 2 == 0:
print("Hot and even")
fallthrough
default:
print("Temperature out of range or odd")
}
스위프트의 switch 구문에서 break는 거의 사용되지 않지만, default에서 아무런 작업도 할 필요가 없는 경우에 유용하다.
... default:
break
}
-알라딘 eBook <핵심만 골라 배우는 SwiftUI 기반의 iOS 프로그래밍> (닐 스미스 지음, 황반석 옮김) 중에서