[android : kotlin] 코틀린 더블콜론(::) 사용 방법 & 사용 예시
코틀린 더블콜론(::)
코틀린에서 변수나 클래스명 앞에 더블콜론(::)을 명시하면 변수에 대한에 속성을 참조할 수 있습니다. 더블콜론을 명시하면 변수가 아닌 객체로 액세스할 수 있기 때문입니다.
함수(메서드) 내에서 변수는 더블콜론으로 명시하여 참조할 수 없습니다. 전역변수나 클래스 등을 더블콜론으로 명시하여 참조하여 사용합니다.
아래 코드를 보면 이해가 빠를 것 같아요.
var x = 1024 fun main(args: Array<String>) { println(::x.get()) println(::x.name) }
[실행 결과]
1024 x
함수(메서드)를 참조하는 코드를 살펴봅니다.
fun isOdd(x: Int): Boolean { return x % 2 != 0 } fun isEven(x: Int): Boolean { return x % 2 == 0 } fun main(args: Array<String>) { val numList = listOf<Int>(1, 2, 3, 4, 5, 6, 7, 8, 9, 10) println("홀수값 : ${numList.filter(::isOdd)}") println("짝수값 : ${numList.filter(::isEven)}") }
[실행결과]
홀수값 : [1, 3, 5, 7, 9] 짝수값 : [2, 4, 6, 8, 10]
fun main(args: Array<String>) { val numList = listOf<Int>(1, 2, 3, 4, 5, 6, 7, 8, 9, 10) var tempList = numList.filter { x: Int -> if(x % 2 == 0) true else false } println("짝수값 : ${tempList[0]}") println("짝수값 : ${tempList[1]}") println("짝수값 : ${tempList[2]}") println("짝수값 : ${tempList[3]}") println("짝수값 : ${tempList[4]}") }
[실행결과]
짝수값 : 2 짝수값 : 4 짝수값 : 6 짝수값 : 8 짝수값 : 10
fun main(args: Array<String>) { val strs = listOf("a", "bc", "def") println(strs.map(String::length)) }
[실행결과]
[1, 2, 3]
아래 또 다른 코드를 봅니다.
lateinit 키워드를 사용하여 초기화한 mediaPlayer 변수가 초기화 되었는지 확인 후 사용해야하겠지요? 아래 코드 스니펫에서 보는 것처림 ::(콜론2개)를 통해서만 접근이 가능해요. 그래야만 isInitialized를 사용하여 체크할 수 있습니다.
package edu.kotlin.study import android.media.MediaPlayer import android.os.Bundle import androidx.appcompat.app.AppCompatActivity class MainActivity : AppCompatActivity() { private lateinit var mediaPlayer: MediaPlayer override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) mediaPlayer = MediaPlayer.create(this, R.raw.bts_dynamite) if (::mediaPlayer.isInitialized) { mediaPlayer.start() } } }
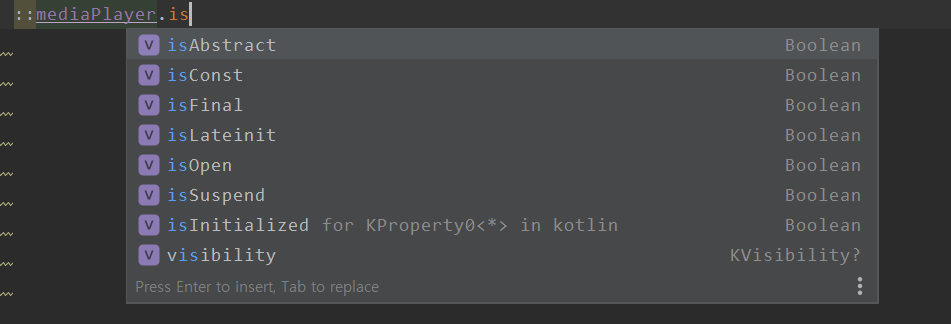
코틀린에서 더블콜론은 리플렉션(Reflection)을 위해 사용됩니다. Reflection은 런타임에 자체 프로그램의 구조를 조사 할 수있는 언어 및 라이브러리 기능 집합입니다.
자바 클래스를 인자로 넘기기 위해 클래스 레퍼런스 타입으로 처리합니다. 코틀린의 클래스 레퍼런스는 “클래스명::class“로 표현합니다. 자바의 클래스 레퍼런스는 “클래스명:class.java“로 표현합니다.
인텐트(Intent) 클래스를 열어보면 인자로 선언한 부분에 Class<?> cls로 받고 있습니다.
/** * Create an intent for a specific component. All other fields (action, data, * type, class) are null, though they can be modified later with explicit * calls. This provides a convenient way to create an intent that is * intended to execute a hard-coded class name, rather than relying on the * system to find an appropriate class for you; see {@link #setComponent} * for more information on the repercussions of this. * * @param packageContext A Context of the application package implementing * this class. * @param cls The component class that is to be used for the intent. * * @see #setClass * @see #setComponent * @see #Intent(String, android.net.Uri , Context, Class) */ public Intent(Context packageContext, Class<?> cls) { mComponent = new ComponentName(packageContext, cls); }
이러한 경우, 자바 클래스를 받으려면 클래스명::class.java로 접근합니다.
var intent = Intent(this, MainActivity::class.java)
[REFERENCE]
kotlinlang.org/docs/reference/reflection.html#reflection
[android : kotlin] 코틀린 lateinit, lazy 사용방법 및 예제