[kotlin]코틀린 findViewById() 사용하는 방법(Java랑 다르다)
코틀린 언어로 findViewById()를 접근하는 방법에 대해 알아보았다. 코틀린언어로 빈액티비티를 선택 후 프로젝트를 하나 생성하였다. 코틀린은 백앤드 코드 확장자가 .kt라는 것을 알게되었다. 코틀린의 약어로 보인다. 여튼 시작은 자바 방식으로 접근하였더니 바로 오류가 터졌다.
코틀린 findViewById()
TextView textview = findViewById(R.id.myTextview);
textview.setText(“Hi~~ Today is beautiful day.”);
위 두 문장 모두 오류가 발생한다. 당연한 결과이다. 자바 언어에 익숙하다보니 코틀린 언어가 조금 낯설다.
코드 모드로 접근하면 아래와 같은 activity_main.xml 레이아웃을 확인할 수 있다.
<?xml version="1.0" encoding="utf-8"?> <androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <TextView android:id="@+id/myTextview" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Hello World!" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintLeft_toLeftOf="parent" app:layout_constraintRight_toRightOf="parent" app:layout_constraintTop_toTopOf="parent" /> </androidx.constraintlayout.widget.ConstraintLayout>
디자인 모드를 클릭 후 왼쪽 하단에 Component Tree에서 TextView를 클릭하면 오른쪽 상단에서 id값을 확인할 수 있다.
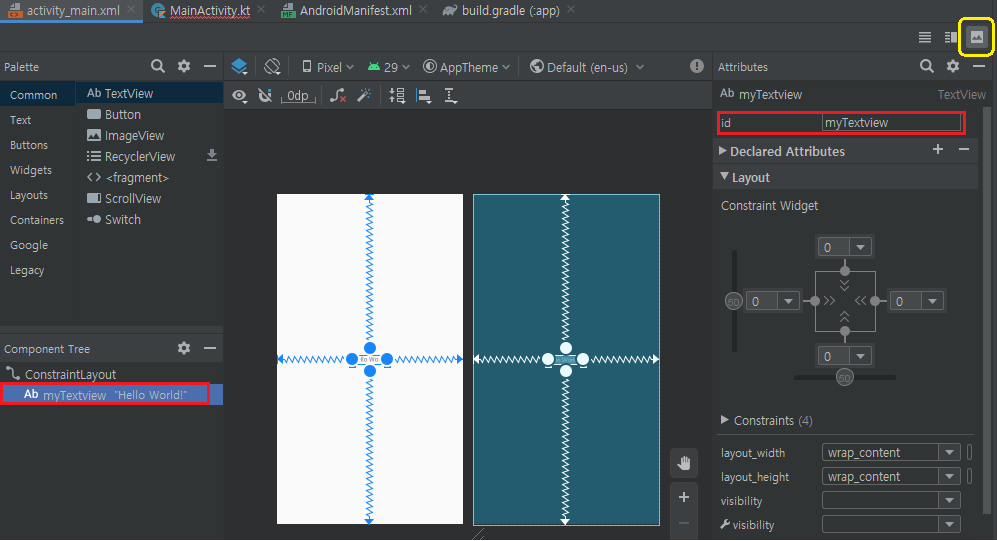
그럼 이제 백앤드 코드에서 접근해보자.
package study.example import androidx.appcompat.app.AppCompatActivity import android.os.Bundle import android.widget.TextView class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) //JAVA에서 접근하는 방법 TextView textview = findViewById(R.id.myTextview); textview.setText("Hi~~ Today is beautiful day."); //코틀린에서 접근하는 방법 val myText : TextView = findViewById(R.id.myTextview) myText.text = "Hi~~ Today is beautiful day." } }
코틀린은 세미콜론(;)을 사용하지 않고 변수 선언 뒤에 변수 타입을 선언한다. 코틀린에서는 findViewById()로 접근 후 변수에 담으려면 val 이나 var로 먼저 선언 후 내가 만들고 싶은 문자를 적용 후 콜론(:)을 찍은 후 변수 타입으로 TextView로 선언 후에 접근이 가능했다. val은 자바에서 final과 동일하다는 것도 알게 되었다. var로 선언하면 변수의 값을 바꿀 수 있다. 하지만 여기서는 var로 선언해야할 이유가 없다. 코틀린에서 View를 닮을 변수를 선언할 때 var과 val 키워드를 사용할 수 있다. 여기서 var은 변경할 수 있는 속성(mutable), val은 변경할 수 없는 속성(immutable)을 뜻한다. var 키워드는 자바에서 일반 변수 선언에 해당하며, final 키워드를 통해 불변 값으로 만드는 것이 val 키워드라고 보면 된다.
써프라이즈하게도 코틀린에서는 더이상 findViewById() 메소드를 사용할 필요가 없어졌다. View의 id값으로 접근이 가능하다. 이것은 정말 획기적이다. 작성해야할 코드가 확 줄어들고 귀찮은 작업이 없어진 것이다. 이런 어메이징한 경우를 보았나……..
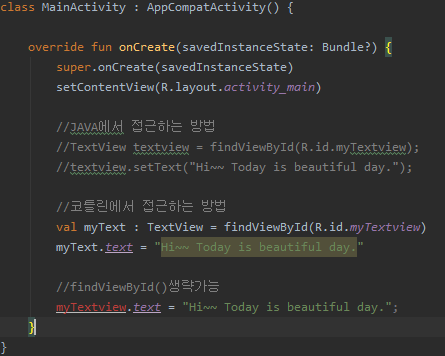
TextView의 id값인 myTextView로 바로 접근이 가능하다. 빨간색으로 오류를 표시해주고 있다. alt+shift +enter 키를 누르거나 마우스 커서를 올려서 import할 수 있다. findViewById()를 사용하지 않는대신 import를 해주는 것이다. 자동 임포트를 하면 소스 코드 상단에 import kotlinx.android.synthetic.main.activity_main.* 가 추가가 된다.
package study.example import androidx.appcompat.app.AppCompatActivity import android.os.Bundle import android.widget.TextView import kotlinx.android.synthetic.main.activity_main.* class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) //코틀린에서 접근하는 방법 val myText : TextView = findViewById(R.id.myTextview) myText.text = "Hi~~ Today is beautiful day." //코틀린 findViewById()생략 가능 myTextview.text = "Hi~~ Today is beautiful day."; } }
자바에서 사용하던 방식으로 하면 textView에 값을 지정하려면 setText()메소드를 사용해야했지만 코틀린은 이것 마져도 줄였다. just 그냥 text접근하면 알아서 setText()메소드를 사용한 효과를 보여준다. text에 마우스 커서를 올리고 ctrl +마우스 왼쪽 버튼을 클릭하면 setText()메소드로 연결됨을 확인할 수 있다.

[REFERENCE]
Kotlin 프로그래밍 언어 알아보기 – developers
androideveryday.com/2018/11/08/findviewbyid-in-kotlin/
velog.io/@jojo_devstory/Kotlin-val-var%EC%9D%98-%EC%B0%A8%EC%9D%B4%EC%A0%90