[android : kotlin] 코틀린 JSON 객체 파싱하는 방법 및 RecyclerView 아이템 추가 및 삭제방법 : Assets폴더 내에 json파일 파싱방법
코틀린 JSON 객체 파싱하는 방법
Assets폴더에 있는 확장자가 json인 파일을 읽어서 파싱 처리 후 리사이클러뷰(RecyclerView)에 추가하는 방법에 대해 알아봅니다. 리사이클러뷰(RecyclerView)에 대한 기본적인 사용방법 및 Assets폴더를 생성하는 방법은 포스팅 하단에 연관글을 참고하세요.
메인액티비티(activity_main.xml) 레이아웃에 RecyclerView를 추가하였습니다.
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical"> <TextView android:id="@+id/textView1" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_margin="10dp" android:gravity="center" android:text="assets폴더에 있는 JSON파일 파싱 예제" android:textAppearance="@style/TextAppearance.AppCompat.Display1" /> <androidx.recyclerview.widget.RecyclerView android:id="@+id/recycler_view" android:layout_below="@+id/textView1" android:layout_width="match_parent" android:layout_height="match_parent" android:layout_above="@+id/bottom_layout"/> <LinearLayout android:id="@+id/bottom_layout" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_alignParentBottom="true" android:layout_margin="10dp" android:orientation="horizontal"> <Button android:id="@+id/del_btn" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_weight="1" android:text="삭제" /> <Button android:id="@+id/add_btn" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_weight="1" android:text="추가" /> </LinearLayout> </RelativeLayout>
리사이클러뷰에서 사용될 아이템뷰 레이아웃(view_item_layout.xml)은 아래와 같습니다.
<?xml version="1.0" encoding="utf-8"?> <androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="60dp" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:background="@color/colorPrimary" android:layout_margin="5dp"> <ImageView android:id="@+id/userImg" android:layout_width="54dp" android:layout_height="54dp" android:layout_marginBottom="4dp" android:layout_marginStart="8dp" android:layout_marginTop="4dp" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" app:srcCompat="@mipmap/ic_launcher_round" /> <TextView android:id="@+id/userNameTxt" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginStart="15dp" android:textSize="20sp" android:textStyle="bold" android:textColor="#FFFFFFFF" app:layout_constraintStart_toEndOf="@+id/userImg" app:layout_constraintTop_toTopOf="@+id/userImg" tools:text="홍길동"/> <TextView android:id="@+id/payTxt" android:layout_width="wrap_content" android:layout_height="wrap_content" android:textSize="17sp" android:textColor="#FFFFFFFF" app:layout_constraintBottom_toBottomOf="@+id/userImg" app:layout_constraintStart_toStartOf="@+id/userNameTxt" tools:text="연봉" /> <TextView android:id="@+id/addressTxt" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginStart="15dp" android:textSize="17sp" android:textColor="#FFFFFFFF" app:layout_constraintBottom_toBottomOf="@+id/payTxt" app:layout_constraintStart_toEndOf="@+id/payTxt" app:layout_constraintTop_toTopOf="@+id/payTxt" tools:text="주소" /> </androidx.constraintlayout.widget.ConstraintLayout>
데이터를 담을 Value Object 클래스를 하나 생성합니다.
package edu.kotlin.study /*dataVo.kt*/ class DataVo(val name: String, val id: String, val address: String, val pay: Int, val photo: String)
리사이클러뷰 어댑터에 현재 클릭한 위치의 position 값을 컨트롤 할 수 있도록 메서드 2개를 추가하였습니다.. 그리고 아이템 추가 및 삭제 메서드를 추가하였습니다. 그리고 onBindViewHolder 메서드를 오버라이드 하는 부분에 setOnClickListener() 메서드를 클릭했을 때 클릭한 아이템의 position를 set 하는 메서드 setPosition()를 호출하였습니다.
데이터를 신규 추가하거나 삭제한 경우 반드시 notifyDataSetChanged()메소드를 호출하여 adapter에게 값이 변경되었음을 알려주어야 리스트가 갱신됩니다.
var mPosition = 0 fun getPosition(): Int { return mPosition } fun setPosition(position: Int) { mPosition = position } fun addItem(dataVo: DataVo) { dataList.add(dataVo) //갱신처리 반드시 해야함 notifyDataSetChanged() } fun removeItem(position: Int) { if (position > 0) { dataList.removeAt(position) //notifyItemRemoved(position) //갱신처리 반드시 해야함 notifyDataSetChanged() } } override fun onBindViewHolder(holder: ItemViewHolder, position: Int) { holder.bind(dataList[position], context) holder.itemView.setOnClickListener { view -> setPosition(position) //Toast.makeText(view.context, "$position 아이템 클릭!", Toast.LENGTH_SHORT).show() } }
리사이클러뷰 CustomAdapter.kt 전체 코드입니다.
package edu.kotlin.study import android.content.Context import android.view.LayoutInflater import android.view.View import android.view.ViewGroup import android.widget.ImageView import android.widget.TextView import android.widget.Toast import androidx.recyclerview.widget.RecyclerView class CustomAdapter( private val context: Context, private val dataList: ArrayList<DataVo> ) : RecyclerView.Adapter<CustomAdapter.ItemViewHolder>() { var mPosition = 0 fun getPosition(): Int { return mPosition } private fun setPosition(position: Int) { mPosition = position } fun addItem(dataVo: DataVo) { dataList.add(dataVo) //갱신처리 반드시 해야함 notifyDataSetChanged() } fun removeItem(position: Int) { if (position > 0) { dataList.removeAt(position) //notifyItemRemoved(position) //갱신처리 반드시 해야함 notifyDataSetChanged() } } inner class ItemViewHolder(itemView: View) : RecyclerView.ViewHolder(itemView) { private val userPhoto = itemView.findViewById<ImageView>(R.id.userImg) private val userName = itemView.findViewById<TextView>(R.id.userNameTxt) private val userPay = itemView.findViewById<TextView>(R.id.payTxt) private val userAddress: TextView = itemView.findViewById<TextView>(R.id.addressTxt) fun bind(dataVo: DataVo, context: Context) { if (dataVo.photo != "") { val resourceId = context.resources.getIdentifier(dataVo.photo, "drawable", context.packageName) if (resourceId > 0) { userPhoto.setImageResource(resourceId) } else { userPhoto.setImageResource(R.mipmap.ic_launcher_round) } } else { userPhoto.setImageResource(R.mipmap.ic_launcher_round) } //TextView에 데이터 세팅 userName.text = dataVo.name userPay.text = dataVo.pay.toString() userAddress.text = dataVo.address } } override fun onCreateViewHolder(parent: ViewGroup, viewType: Int): ItemViewHolder { val view = LayoutInflater.from(context).inflate(R.layout.view_item_layout, parent, false) return ItemViewHolder(view) } override fun onBindViewHolder(holder: ItemViewHolder, position: Int) { holder.bind(dataList[position], context) holder.itemView.setOnClickListener { view -> setPosition(position) Toast.makeText(view.context, "$position 아이템 클릭!", Toast.LENGTH_SHORT).show() } holder.itemView.setOnLongClickListener { view -> setPosition(position) Toast.makeText(view.context, "$position 아이템 롱클릭!", Toast.LENGTH_SHORT).show() return@setOnLongClickListener true } } override fun getItemCount(): Int { return dataList.size } }
assets.open()메서드를 사용하여 assets폴더에 있는 json파일의 내용을 읽어와 문자열로 처리후 JSONObject를 사용하여 문자열 데이터를 JSONObject 객체로 변환합니다.
try { val inputStream = assets.open("sample_code.json") val size = inputStream.available() val buffer = ByteArray(size) inputStream.read(buffer) inputStream.close() var strJson = String(buffer, Charsets.UTF_8) val jsonObject = JSONObject(strJson) //파일 읽어오는 IO처리 부분 메서드 분기 처리방법 val jsonObject2 = JSONObject(loadJSONFileFromAsset()) val userArray = jsonObject.getJSONArray("usersInfo") for (i in 0 until userArray.length()) { val baseInfo = userArray.getJSONObject(i) val detail = baseInfo.getJSONObject("detail") val tempData = DataVo( baseInfo.getString("name"), baseInfo.getString("id"), detail.getString("address"), detail.getInt("pay"), detail.getString("photo") ) userList.add(tempData) } } catch (e: JSONException) { e.printStackTrace() } catch (e: IOException) { e.printStackTrace() }
메인액티비티클래스(MainActivity.kt) 전체 코드 내용입니다.
package edu.kotlin.study import android.annotation.SuppressLint import android.media.MediaPlayer import android.os.Bundle import android.webkit.* import android.widget.ProgressBar import androidx.appcompat.app.AppCompatActivity import androidx.recyclerview.widget.LinearLayoutManager import kotlinx.android.synthetic.main.activity_main.* import org.json.JSONException import org.json.JSONObject import java.io.IOException import java.nio.charset.Charset class MainActivity : AppCompatActivity() { private var userList: ArrayList<DataVo> = ArrayList() @SuppressLint("SetJavaScriptEnabled") override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) try { val inputStream = assets.open("sample_code.json") val size = inputStream.available() val buffer = ByteArray(size) inputStream.read(buffer) inputStream.close() var strJson = String(buffer, Charsets.UTF_8) val jsonObject = JSONObject(strJson) //파일 읽어오는 IO처리 부분 메서드 분기 처리방법 val jsonObject2 = JSONObject(loadJSONFileFromAsset()) val userArray = jsonObject.getJSONArray("usersInfo") for (i in 0 until userArray.length()) { val baseInfo = userArray.getJSONObject(i) val detail = baseInfo.getJSONObject("detail") val tempData = DataVo( baseInfo.getString("name"), baseInfo.getString("id"), detail.getString("address"), detail.getInt("pay"), detail.getString("photo") ) userList.add(tempData) } } catch (e: JSONException) { e.printStackTrace() } catch (e: IOException) { e.printStackTrace() } val mAdapter = CustomAdapter(this, userList) recycler_view.adapter = mAdapter val layout = LinearLayoutManager(this) recycler_view.layoutManager = layout recycler_view.setHasFixedSize(true) add_btn.setOnClickListener { mAdapter.addItem(DataVo("임시 추가", "test14", "광주시", 32000000, "user_img_03")) // notifyDataSetChanged를 호출하여 adapter의 값이 변경되었다는 것을 알려준다. //mAdapter.notifyDataSetChanged() } del_btn.setOnClickListener { mAdapter.removeItem(mAdapter.getPosition()) // notifyDataSetChanged를 호출하여 adapter의 값이 변경되었다는 것을 알려준다. //mAdapter.notifyDataSetChanged() } } private fun loadJSONFileFromAsset(): String { return try { val inputStream = assets.open("users_list.json") val size = inputStream.available() val buffer = ByteArray(size) //val charset: Charset = Charsets.UTF_8 inputStream.read(buffer) inputStream.close() String(buffer, Charsets.UTF_8) } catch (ex: IOException) { ex.printStackTrace() return "" } } }
[build.gradle(Module:app)]
apply plugin: 'com.android.application' apply plugin: 'kotlin-android' apply plugin: 'kotlin-android-extensions' android { compileSdkVersion 29 defaultConfig { applicationId "edu.kotlin.study" minSdkVersion 22 targetSdkVersion 29 versionCode 1 versionName "1.0" testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner" } buildTypes { release { minifyEnabled false proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro' } } } dependencies { implementation fileTree(dir: "libs", include: ["*.jar"]) implementation "org.jetbrains.kotlin:kotlin-stdlib:$kotlin_version" implementation 'androidx.core:core-ktx:1.3.1' implementation 'androidx.appcompat:appcompat:1.2.0' implementation 'androidx.constraintlayout:constraintlayout:2.0.1' implementation 'androidx.legacy:legacy-support-v4:1.0.0' implementation 'androidx.recyclerview:recyclerview:1.1.0' testImplementation 'junit:junit:4.12' androidTestImplementation 'androidx.test.ext:junit:1.1.2' androidTestImplementation 'androidx.test.espresso:espresso-core:3.3.0' }
[build.gradle(Project)]
// Top-level build file where you can add configuration options common to all sub-projects/modules. buildscript { ext.kotlin_version = "1.3.72" repositories { google() jcenter() } dependencies { classpath "com.android.tools.build:gradle:4.0.1" classpath "org.jetbrains.kotlin:kotlin-gradle-plugin:$kotlin_version" // NOTE: Do not place your application dependencies here; they belong // in the individual module build.gradle files } } allprojects { repositories { google() jcenter() } } task clean(type: Delete) { delete rootProject.buildDir }
[실행화면]
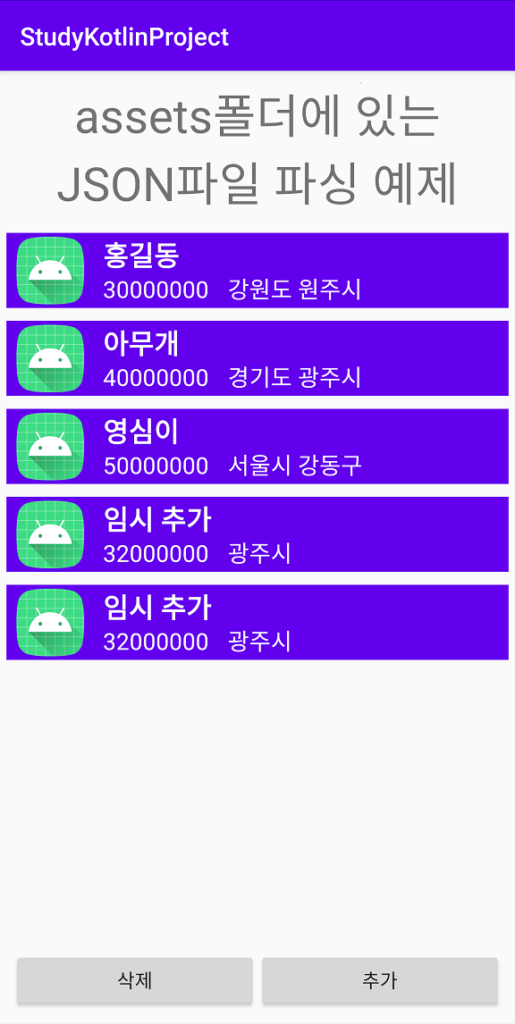
[REFERENCE]
[연관글]
[android : kotlin] 코틀린 RecyclerView 사용방법 및 예제
[android : kotlin] 코틀린 RecyclerView 아이템 추가 및 삭제하는 방법
[추천 도구]
온라인 JSON 포맷터ONLINE JSON FORMATTER